Finance Tracker
Finance Tracker
A full-stack finance tracker built with Next.js, Hono.js, Drizzle, Clerk, and Tailwind. Features include income and expense tracking, CRUD operations, transaction tables, and charts for data visualization.
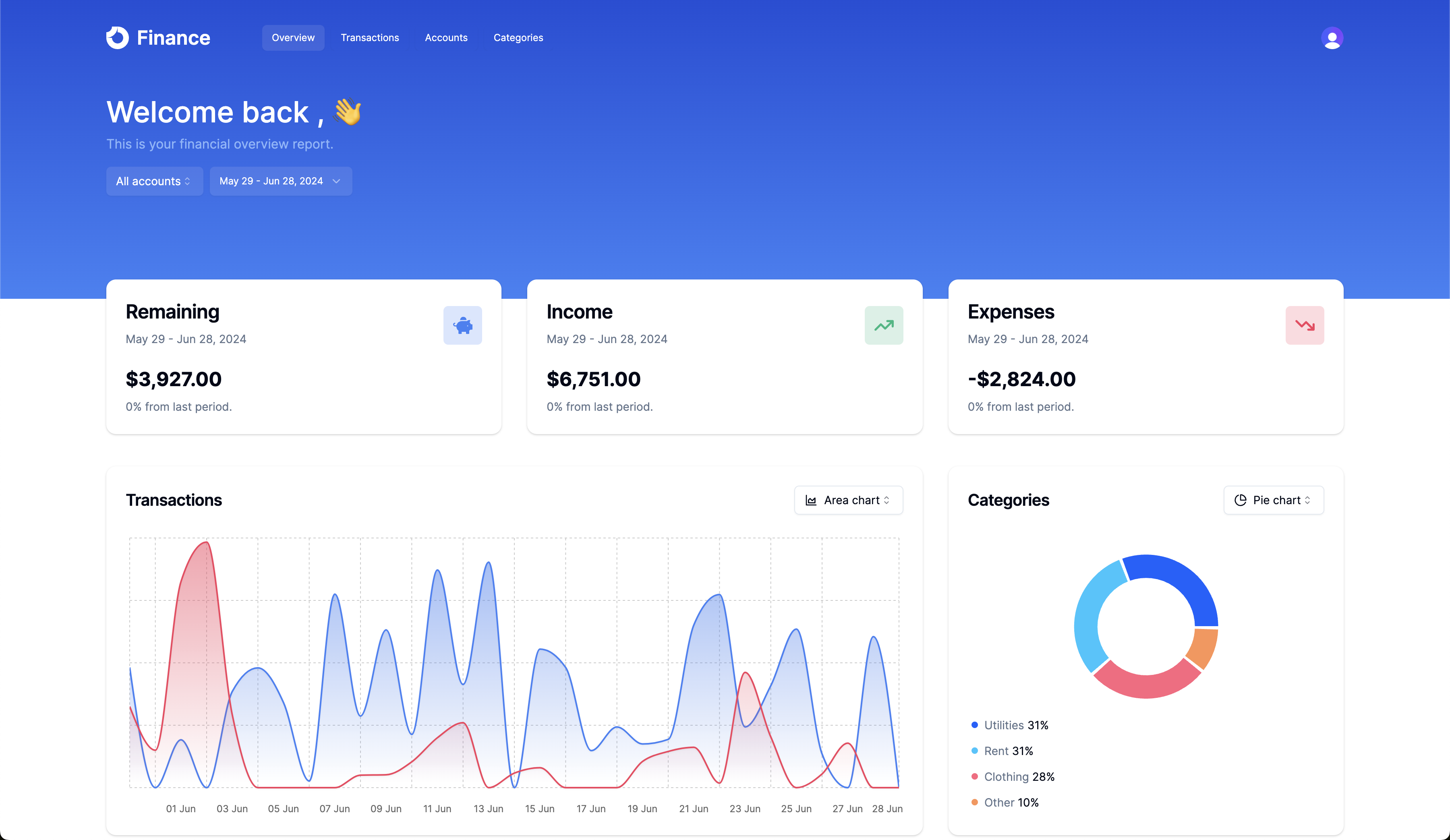
Overview
This is a full-stack finance tracking website built using modern technologies for a seamless and efficient user experience. The website leverages Next.js for the frontend, Hono.js with Next.js for the backend, Drizzle for ORM, Clerk for authentication, and Tailwind Shadcn UI for styling. The primary functionality includes tracking income and expenses with features like CRUD operations for categories and transactions, and listing transactions with tables and various charts (pie, area, line, etc.).
Technologies Used
- Frontend:Next.js
- Backend:Hono.js with Next.js
- ORM:Drizzle
- Authentication:Clerk
- Styling:Tailwind Shadcn UI
Main Features
- CRUD Operations for Categories and Transactions
- List Transactions:Display transactions in a table format
- Charts:Visualize transactions using pie, area, and line charts
Development Process
Clerk Integration
- Set up Clerk for authentication.
- Implement Clerk middleware in Hono.js.
Backend Development with Hono.js
- Account Post:
- Utilize Drizzle Zod for schema validation.
- Implement Hono zValidator middleware for request validation.
- Create POST endpoint for accounts.
- Transactions Schema:
- Define schema with fields such as id, amount (integer, rounded to three decimal places), and payee.
- Establish relationships with accounts and categories.
- Cascade delete transactions when an account is deleted.
- Set categoryId to null when a category is deleted.
Frontend Development with Next.js
- Header Component:Create a reusable header component.
- Sheet Component:Implement
useMountedState
hook for state management. - Zustand:Utilize Zustand for global state management.
Data Management
- React Query:
- Implement mutations for creating accounts.
- Refresh account list upon mutation.
User Interface
- Data Table:Create a data table for listing accounts and transactions.
- Edit Account Form:Implement form for editing accounts.
- Account Bulk Deletes:Enable bulk deletion of accounts.
API Development
- Categories API + UI:Mirror the functionality of accounts for categories.
- Transactions API:
- Implement GET, POST, PATCH, DELETE endpoints for transactions.
- Define Zod query for filtering transactions.
- Utilize joins to fetch related data for categories and accounts.
Transaction Management
- Hooks:Create custom hooks for managing transactions.
- Transactions Form:Develop a form for adding and editing transactions.
- Transactions Page:Design a page to list and manage transactions.
- CSV Import: Implement CSV import functionality using
react-papaparse
.- Default to list mode.
- Transition to import mode and pass data to
ImportCard
. - Use bulk create for batch importing transactions.
Dashboard
- Summary API:Implement an API for calculating summary data for graphs.
- Dashboard Cards:Display total income and total expense using data grids.
- Charts:Create pie, area, and line charts for visualizing transaction data.
- Filters:Add date and account filters for refined data visualization.
Deployment
- Deploy the application using Vercel.
- Purchase and configure the domain
namk.dev
. - Upgrade to Vercel Pro for enhanced features.
Notes
- Ensure all transactions are listed for the last 30 days by default.
- Implement a demo account for user testing.
- Organize components logically for maintainability.
This documentation provides a comprehensive overview of the finance tracking website, detailing the technologies used, main features, development process, and key notes for deployment and user experience. This should serve as a guide for understanding the project and its functionalities.